Mimesis - Fake Data Generator

Description
Mimesis is a high-performance fake data generator for Python, which provides data for a variety of purposes in a variety of languages. The fake data could be used to populate a testing database, create fake API endpoints, create JSON and XML files of arbitrary structure, anonymize data taken from production and etc.
The key features are:
- Performance: The fastest data generator available for Python.
- Extensibility: You can create your own data providers and use them with Mimesis.
- Generic data provider: The simplified access to all the providers from a single object.
- Multilingual: Supports data for a lot of languages.
- Data variety: Supports a lot of data providers for a variety of purposes.
- Schema-based generators: Provides an easy mechanism to generate data by the schema of any complexity.
- Country-specific data providers: Provides data specific only for some countries.
Installation
To install mimesis, simply use pip:
[venv] ~ ⟩ pip install mimesis
Usage
This library is really easy to use and everything you need is just import an object which represents a type of data you need (we call such object Provider).
In example below we import provider Person, which represents data related to personal information, such as name, surname, email and etc:
>>> from mimesis import Person
>>> person = Person('en')
>>> person.full_name()
'Brande Sears'
>>> person.email(domains=['mimesis.name'])
'[email protected]'
>>> person.email(domains=['mimesis.name'], unique=True)
'[email protected]'
>>> person.telephone(mask='1-4##-8##-5##3')
'1-436-896-5213'
More about the other providers you can read in our documentation.
Locales
Mimesis currently includes support for 34 different locales. You can specify a locale when creating providers and they will return data that is appropriate for the language or country associated with that locale.
Let's take a look how it works:
>>> from mimesis import Person
>>> from mimesis.enums import Gender
>>> de = Person('de')
>>> en = Person('en')
>>> de.full_name(gender=Gender.FEMALE)
'Sabrina Gutermuth'
>>> en.full_name(gender=Gender.MALE)
'Layne Gallagher'
Providers
Mimesis support over twenty different data providers available, which can produce data related to people, food, computer hardware, transportation, addresses, internet and more.
See API Reference for more info.
The data providers are heavy objects since each instance of provider keeps in memory all the data from the provider's JSON file so you should not construct too many providers.
Generating structured data
You can generate dictionaries which can be easily converted to any the format you want (JSON/XML/YAML etc.) with any structure you want.
Let's build dummy API endpoint, using Flask to illustrate the idea:
from flask import Flask, jsonify, request
from mimesis.schema import Field, Schema
from mimesis.enums import Gender
app = Flask(__name__)
@app.route('/apps', methods=('GET',))
def apps_view():
locale = request.args.get('locale', default='en', type=str)
count = request.args.get('count', default=1, type=int)
_ = Field(locale)
schema = Schema(schema=lambda: {
'id': _('uuid'),
'name': _('text.word'),
'version': _('version', pre_release=True),
'timestamp': _('timestamp', posix=False),
'owner': {
'email': _('person.email', domains=['test.com'], key=str.lower),
'token': _('token_hex'),
'creator': _('full_name', gender=Gender.FEMALE)},
})
data = schema.create(iterations=count)
return jsonify(data)
Below, on the screenshot, you can see a response from this fake API (/apps
):
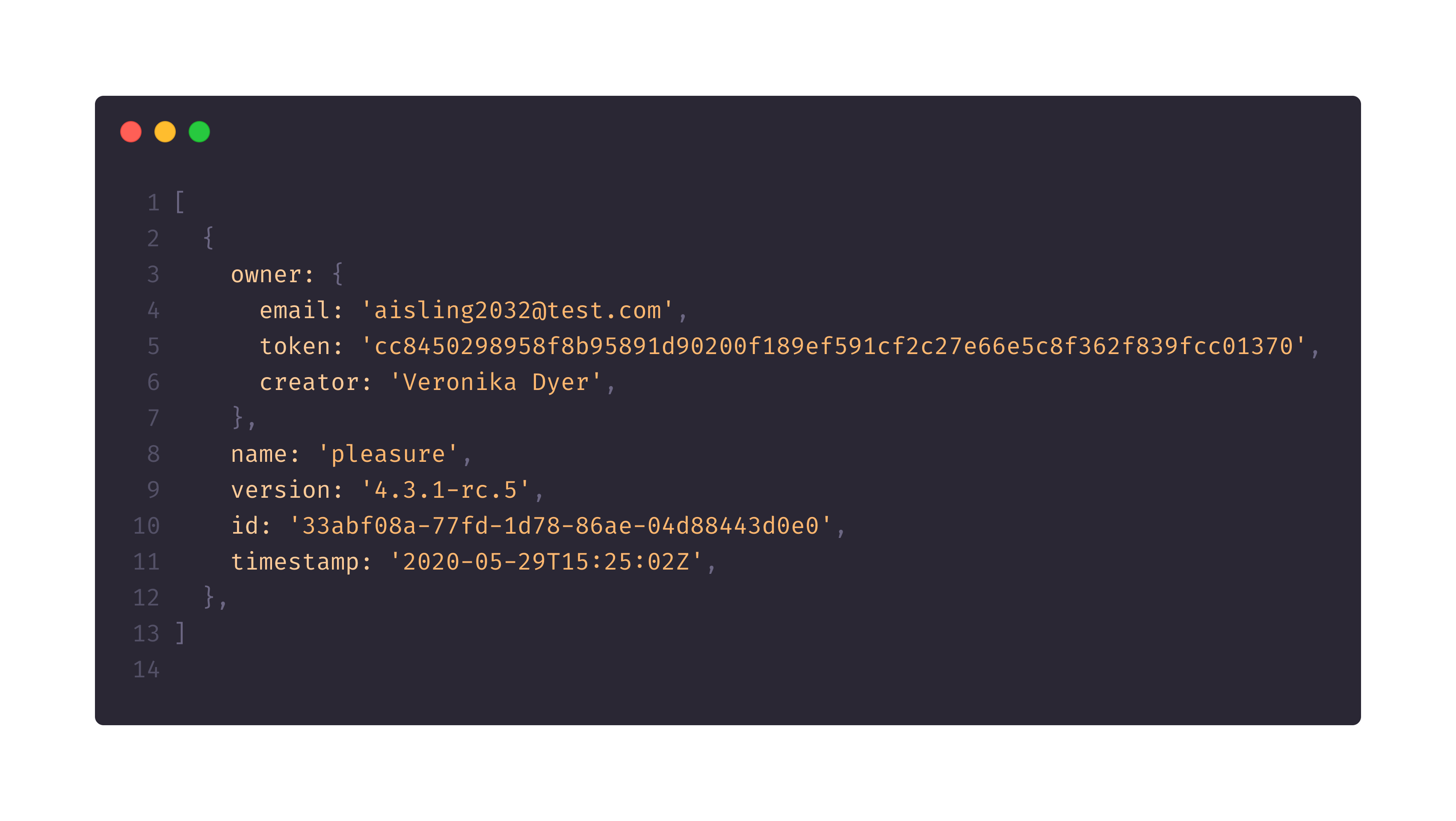
See Schema and Fields for more info.
Documentation
You can find the complete documentation on the Read the Docs.
It is divided into several sections:
You can improve it by sending pull requests to this repository.
How to Contribute
- Take a look at contributing guidelines.
- Check for open issues or open a fresh issue to start a discussion around a feature idea or a bug.
- Fork the repository on GitHub to start making your changes to the your_branch branch.
- Add yourself to the list of contributors.
- Send a pull request and bug the maintainer until it gets merged and published.
Thanks
Supported by JetBrains.
Disclaimer
The authors of Mimesis do not assume any responsibility for how you use it or how you use data generated with it. This library was designed with good intentions to make testing easier. Do not use the data generated with Mimesis for illegal purposes.
License
Mimesis is licensed under the MIT License. See LICENSE for more information.